Test SAM Server
Thus far, you have deployed it, so it can now be tested.
For testing, you can use the sam-example-client in the Talend ESB examples.
The file agent.properties:
collector.scheduler.interval=500
collector.maxEventsPerCall=10
log.messageContent=true
log.maxContentLength=-1
service.url=http://localhost:8040/services/MonitoringServiceSOAP
service.retry.number=3
service.retry.delay=5000
Spring configuration file contents:
<import resource="classpath:META-INF/cxf/cxf.xml" />
<import resource="classpath:META-INF/cxf/cxf-extension-soap.xml" />
<import resource="classpath:META-INF/cxf/cxf-servlet.xml" />
<import resource="classpath:META-INF/tesb/agent-context.xml" />
<bean id="fixedProperties"
class="org.talend.esb.sam.common.handler.impl.CustomInfoHandler">
<property name="customInfo">
<map>
<entry key="Application name" value="Client" />
</map>
</property>
</bean>
<bean class="org.talend.esb.sam.examples.client.CustomerServiceTester">
<property name="customerService" ref="customerService" />
</bean>
<jaxws:client id="customerService"
address="${serviceUrl}"
serviceClass="com.example.customerservice.CustomerService">
<jaxws:features>
<!-- <bean class="org.apache.cxf.feature.LoggingFeature" />-->
<ref bean="eventFeature"/>
</jaxws:features>
</jaxws:client>
Then provide the following code:
// read configuration
System.setProperty("serviceUrl",
"http://localhost:9090/CustomerServicePort/CustomerServiceService");
ClassPathXmlApplicationContext context =
new ClassPathXmlApplicationContext("/client.xml");
CustomerServiceTester tester = context.getBean(CustomerServiceTester.class);
tester.testCustomerService();
As you see, the value of "serviceUrl" is sent with service URL, which will fill the contents of bean "customerService". Now you willl turn to the CustomerServiceTester to do a real test:
public void testCustomerService() throws NoSuchCustomerException {
List<Customer> customers = null;
// First we test the positive case where customers are found and we retrieve
// a list of customers
System.out.println("Sending request for customers named Smith");
customers = customerService.getCustomersByName("Smith");
System.out.println("Response received");
Assert.assertEquals(2, customers.size());
Assert.assertEquals("Smith", customers.get(0).getName());
try {
Thread.sleep(1000);
} catch (InterruptedException e1) {
}
// Then we test for an unknown Customer name
// and expect a NoSuchCustomerException
try {
customers = customerService.getCustomersByName("None");
Assert.fail("We should get a NoSuchCustomerException here");
} catch (NoSuchCustomerException e) {
System.out.println(e.getMessage());
Assert.assertNotNull("FaultInfo must not be null", e.getFaultInfo());
Assert.assertEquals("None", e.getFaultInfo().getCustomerName());
System.out.println("NoSuchCustomer exception was received as expected");
}
System.out.println("All calls were successful");
}
As you can see, the test consisted in requesting a customer. Every time a test is executed, a log message will be recorded into the database you configured in "org.talend.esb.sam.server.cfg". A database viewer can be used to confirm that, for example DbVisualizer:
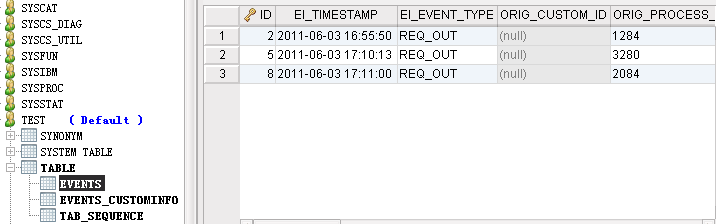
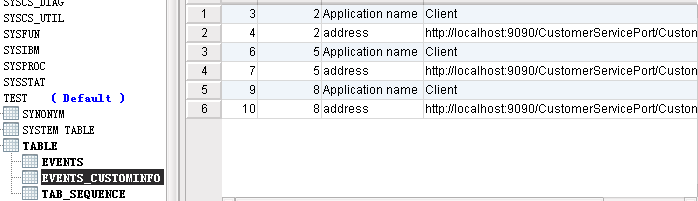